concerning the GirlTech IMME,
utilizing the GoodFET's Chipcon Debugger
to instrument Michael Ossmann's $16 Pocket Spectrum Analyzer.
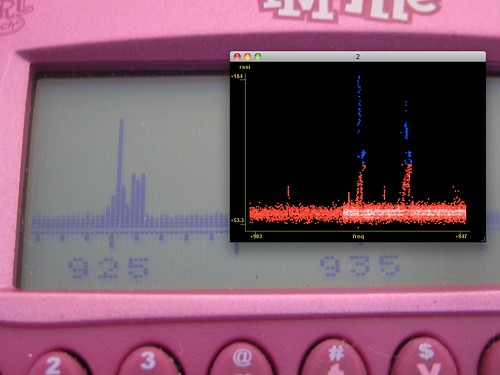
Often a neighbor, such as myself, finds himself with a damned useful tool that's missing logging. Further, when this is a black-box application, it is undeniably inconvenient to patch in logging where no communications method exists. In this brief article, I will demonstrate how to instrument a Chipcon CC1110 application using Python and a GoodFET with zero bytes of modification to the original firmware image. My target's source code is available, but the technique applies just as well to black-box firmware.
Specifically, I want to dump the raw data from Michael Ossmann's $16 Pocket Spectrum Analyzer in order to demo it on stage at our Toorcon 12 talk, Real Men Carry Pink Pagers. I'll assume the reader to be familiar with Mike's article, as well as my article on wiring an IMME for debugging with a GoodFET. Readers wishing to play along can order a GoodFET31 for little or no money by following these instructions.
From the datasheet or Mike's Makefile it can be seen that the external RAM (XDATA, which is called external for historic reasons) section begins at 0xF000. The only structure at this location is xdata channel_info chan_table[NUM_CHANNELS], where NUM_CHANNELS is defined to be 132 and the channel_info struct is eight bytes, defined as
typedef struct {
/* frequency setting */
u8 freq2;
u8 freq1;
u8 freq0;
/* frequency calibration */
u8 fscal3;
u8 fscal2;
u8 fscal1;
/* signal strength */
u8 ss;
u8 max;
} channel_info;
The first several entries in this struct might be as follows. The first of these lines defines the frequency to be 0x22af4b, the frequency calibration to be 0xef2c27, and the signal strength to be 0x41. The final byte defines the maximum strength, which is zero unless max-highlighting mode is turned on.
22 af 4b ef 2c 27 41 00
22 b1 43 ef 2c 27 3e 00
22 b3 3b ef 2c 27 46 00
22 b5 33 ef 2c 27 3c 00
22 b7 2b ef 2c 27 44 00
22 b9 23 ef 2c 28 3c 00
22 bb 1b ef 2c 28 42 00
22 bd 13 ef 2c 28 3f 00
22 bf 0b ef 2c 28 3d 00
22 c1 03 ef 2c 28 40 00
22 c2 fb ef 2c 28 3d 00
22 c4 f3 ef 2c 28 3e 00
...
This information can be extracted by halting and resuming the CPU, just as I did in my article on the PRNG Vulnerability of Z-Stack's ZigBee SEP ECC implementation. That is, GoodFETCC.CCpeekdatabyte() is used to grab these bytes from the CC1110's RAM. The following code dumped the fragment shown above.
#!/usr/bin/env python
import sys;
sys.path.append('/Users/travis/svn/goodfet/trunk/client/')
from GoodFETCC import GoodFETCC;
from intelhex import IntelHex16bit, IntelHex;
import time;
client=GoodFETCC();
client.serInit();
client.setup();
client.start();
bytescount=8*132;
bytestart=0xf000;
while 1:
time.sleep(1);
client.CChaltcpu();
dump="";
for foo in range(0,bytescount):
dump=("%s %02x" % (dump,client.CCpeekdatabyte(bytestart+foo)));
if foo%8==7: dump=dump+"\n";
print dump;
sys.stdout.flush();
client.CCreleasecpu();
To get proper data, it is necessary to add a wake-up delay of a few seconds, so that the table is populated by the first sampling. Additionally, it is handy to convert the frequency to MHz from its native unit (Hz/396.728515625). Finally, successive pages should be separated by a time column in order to facilitate graphing. The result is this script, which can be found in the GoodFET project as "contrib/ccspecantap/specantap.py".
An example recording can be seen below, as a spectrum recording of a friend's Kenwood FreeTalk UBZ-AL14 FM Transceiver unit. This is compatible with other family FM radios, and I wanted to know which center frequencies were in use. In the first image, I've highlighted the noise floor as blue and the peaks as green. In the second image, the time domain is used to show broadcasts on several channels in sequence, all of which fall into one of the two center frequencies: 925MHz and 935MHz. The raw data is here, best viewed with NASA's excellent Viewpoints tool.
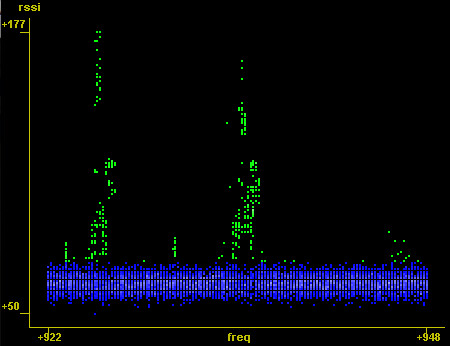

Future additions to this project will include integration into the GoodFET radio framework, as well as a 2.4GHz version built around the CC2430 and CC2530. The performance of the tap can be greatly improved by using block transactions rather than peeking individual bytes, and the view can be re-tuned by poking the configuration IDATA variables, positions of which are described in specan.rst as produced by the SDCC compiler.
It's also handy to know that a Chipcon radio will halt if the 0xA5 opcode is executed. In this manner, patching a single byte of a while() loop can allow the state at every iteration to be dumped.
This technique of scripted debugging through a programmer can be handy for more than instrumentation. Unit tests can be run on an assembly line without additional wiring, and--by single-stepping--execution traces of unknown firmware can be generated for assisting in reverse engineering. Those with enough patience can use this to fuzz test embedded systems for security vulnerabilities.
For help instrumenting your own Chipcon or MSP430 project, join us in #goodfet on irc.freenode.net or send me an email. The project has advanced to the point where interesting things can be done from Python alone, and I'd quite like to see what could be done with it.
33 comments:
Neat trick. Another way to add logging would be to reverse engineer the link to the Cypress chip on the dongle.
http://blog.hodgepig.org/2010/10/22/im-me-usb-dongle-hacking/
My cousin recommended this blog and she was totally right keep up the fantastic work!
calibration company
One thing that always troubled me was that even with the net, most people still don’t tend to address about the topic in this quality.
Structural Analysis
Structural Analysis in USA
Every student has the chance of enjoying our affordable informative research papers. The writers hired at the company are professionals with significant experience.
gmail inloggen ok
حامد برادران دلتنگ
رضا شیری خوش قلب
مازیار فلاحی
مرتضی اشرفی
Best Digital Marketing Company
Digital Marketing Expert
SEO Expert & Company
Website Design and Development Company
Best IT Consultant
Biggest Pharma Company
Thank you for providing such informatic steps regarding sim lock. I have read your blog and share this with my friend they like your blog. The information provided by you is really helpful. you can use Quickbooks software to manage your business taxes call on Quickbooks support number
Thanks for sharing!
Massage Therapist Services
Maid Services in Toronto
Taipan Express Movers is the best service provider for moving high value items, pets, children and more for both local and international locations. We are also offering furniture storage solutions.
Packers and Movers Malaysia to America
Packers and Movers Malaysia to Dubai
Packers and Movers to Sarawak
Packers and Movers to Selangor
Packers and Movers to Terengganu
Packers and Movers to Kuala Lumpur
Packers and Movers to Labuan
Packers and Movers to Putrajaya
Full merchant account is another name for merchant account as such. Merchant account is useful for any business in order to expand its customer base online. With most merchant account providers, the vendor can expect to receive the funds paid by the customer within one or two days. http://www.confiduss.com/en/info/faq/bank-accounts/merchant/full-merchant-account-advantages/
For help instrumenting your own Chipcon or MSP430 project, join us in #goodfet on irc.freenode.net or send me an email. The project has advanced to the point where interesting things can be done from Python alone, and I'd quite like to see what could be done with it. lockets canada , lockets australia
Đặt vé tại phòng vé Aivivu, tham khảo
giá vé máy bay đi Mỹ khứ hồi
vé máy bay giá rẻ đi sài gòn vietjet
vé máy bay từ nha trang đến hà nội
vé máy bay sài gòn cam ranh
vé máy bay đi quy nhơn tháng 12
thuê xe ra sân bay nội bài
combo novotel phú quốc 3 ngày 2 đêm
Aivivu - đại lý chuyên vé máy bay trong nước và quốc tế
Vé máy bay đi Mỹ
giá vé máy bay từ mỹ về việt nam tháng 12
vé máy bay từ canada về việt nam
bay nhật bản việt nam
vé máy bay từ hàn về Việt Nam
Vé máy bay từ Đài Loan về VN
bảng giá khách sạn cách ly tại hà nội
Sometimes you just want to relax at home, but how can one relax if they do not feel comfortable? That is why Dshred is offering the most comfortable Women Loungewear products available. Get our knitted loungewear sets for maximum comfort or our Women’s loungewear tracksuits if you plan to go out for a bit.
Informative blog shared, Keep sharing such blogs.
Python Training in Pune
Informative blog shared, Keep sharing such blogs.
Python Classes in Pune
Buy the latest Lawn Collection from Reign and enjoy the best price with free home delivery.
Informative blog shared, Keep sharing such blogs.
Know more about SAP FICO Course
I recently came across your article and have been reading along. I want to express my admiration of your writing skill and ability to make readers read from the beginning to the end.
Python Training In Pune
Great! Learn from the best Python course in Delhi that offers 200+ courses designed by Industry professionals.
Thanks a lot for sharing this valuable and useful content with us.
สมัคร igoal
Thanks a lot for sharing this valuable and useful content with us.
jual emas
jual emas
wordpress design services agency Need professional WordPress Web Design Services? We're experts in developing attractive mobile-friendly WordPress websites for businesses. Contact us today!
First of all thanks for post such nice stuff. its really valuable contents. Hope you will provide same as in future posts EIS SM Pendrive Classes
That's great admin. Its really valuable information, Thanks for giving these tip & guidance to us. Thanks for sharing such nice stuff. Keep it up Ca Inter Fm Eco Pendrive classes
CA Inter FM & ECO Pendrive Classes by Sumit Parashar Sir For May 23 & Onwards | Complete FM ECO Course | Full HD Video + HQ Sound
An ever increasing number of areas of business are prepared to introduce on-request applications: taxi, back rub, food or products conveyance, clinical benefits, cleaning administrations, etc. In the event that you furnish your clients with on-request benefits, search for an application originator for enlist - your application gets an opportunity to be a major achievement. The combination of the applications on the smartwatches is plausible to improve the way of life of your clients. The client can stay in contact with the administrations and applications without visiting a tablet or telephone. Also, the organizations as of now use it. Wearable applications are the most famous in the clinical circle>> application development team
Proofreading is an integral part of the grammar writing process. Without proper grammar and punctuation, readers may not understand your ideas properly and may not be able to make the most of them. You can use a free online grammar checker to online sentence check and correct spelling mistakes.
I wanted to leave a little comment to support you and wish you the
best of luck. We wish you the best of luck in all of your blogging
endeavors.
Java training institution in Hyderabad
Amazing write-up always finds something interesting.xx python training in pune
Great post, thanks or sharing valuable information, keep us posted more Python Course in Pune best python training institute in Pune
Great article! I found your insights really informative. I would like to share information about the company. Introducing our cutting-edge Grocery App Development Company, where innovation meets convenience! Elevate your grocery shopping experience with our custom-built mobile applications that seamlessly blend technology and user-friendly interfaces.
Post a Comment